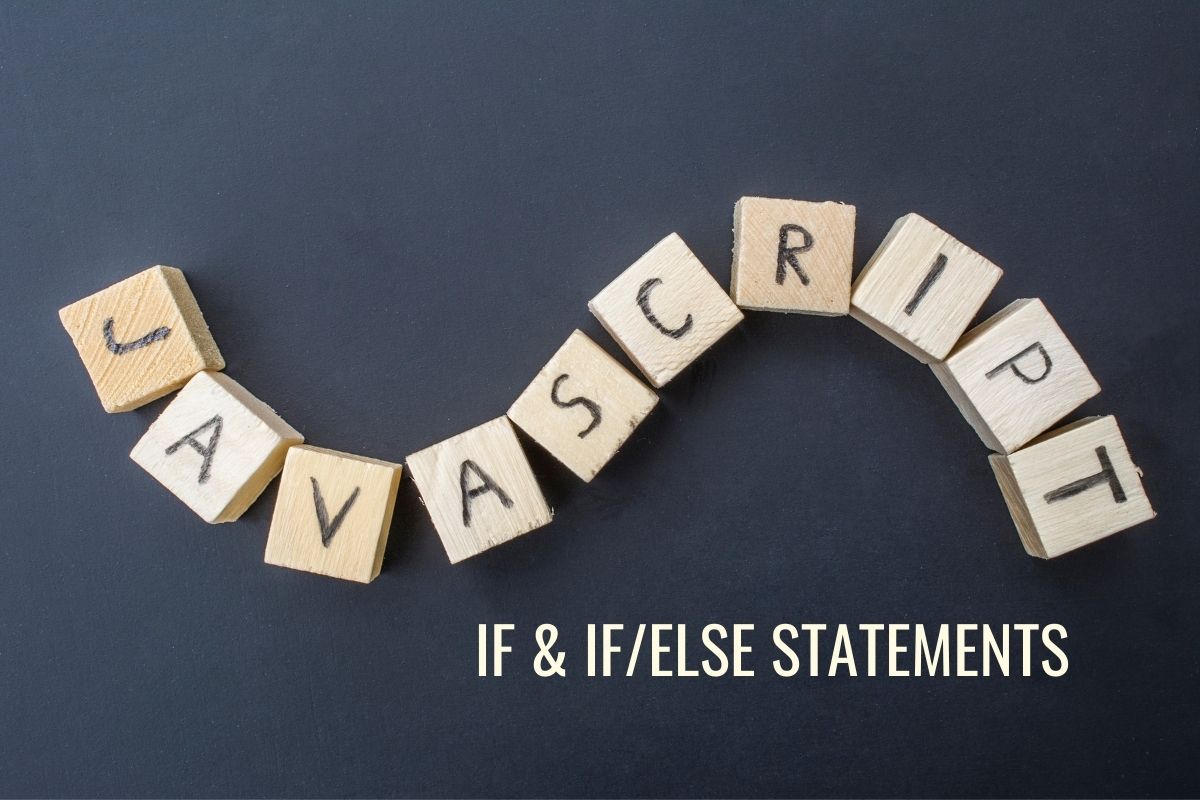
IF and IF/ELSE Statements
An IF statements and IF/ELSE statements are types of control structures in JavaScript. They are used to control the flow of code execution.
IF Statements
IF statements will execute code if an associated condition evaluates to true.
IF statements are made up of three main parts:
- The if keyword
- The condition that evaluates to true or false
- The code block to be executed if the condition evaluates to true
Correct syntax is important. When writing an IF statement, the if keyword is in all lowercase letters. The condition of the IF statement is contained within parentheses, and curly braces are used to contain the code block.
Example 1:
if(averageGrade >= 70){ alert("You pass!"); }
The code block will only be executed, if the condition of the IF statement evaluates to true. If the condition evaluates to false, the code block will not run.
Using the example above, if the value for the averageGrade variable is greater than or equal to 70, the condition evaluates to true. So, the code block will run and the browser will show an alert message saying "You pass!".
However, if the value for the averageGrade variable is less than 70. The condition will evaluate to false and the code block will not run. So, the "You pass!" alert will not show in the browser.
IF/ELSE Statements
IF/ELSE statements are similar to IF statements, but they take control of code execution one step further. Instead of only executing a code block if the condition of the IF statement evaluates to true, IF/ELSE statements are set-up to run a different code block if the condition evaluates to false.
IF/ELSE statements are set up similarly to IF statements. The difference is an ELSE statement is added after the IF statement. An ELSE statement contains two main parts:
- The else keyword
- The code block to be executed if the condition of the IF statement evaluates to false
The ELSE statement starts immediately on the same line after the closing curly brace of the IF statement. The else keyword is in all lowercase letters, and the associated code block is contained within curly braces.
Example 2:
if(averageGrade >= 70){ alert("You pass!"); }else{ alert("Better luck next time."); }
Similar to Example 1, if the value for the averageGrade variable is greater than or equal to 70 in Example 2, the IF statement's condition will evaluate to true. The first code block will run and the "You pass!" alert will show in the browser.
If the value for the averageGrade value is less than 70, the IF statement's condition will again evaluate to false. However, unlike in Example 1 where no code was executed when the condition evaluated to false, this time the second code block (associated with the ELSE statement) will run and the "Better luck next time." alert will show in the browser.